- Book Desciption: This books is Free to download. 'C# 4.0 in a Nutshell The Definitive Reference Book book' is available in PDF Formate. Learn from this free book and enhance your skills.
- 4.0 Programming Black Book is the one-time reference book, written from the programmers point of view, containing hundreds of examples covering every aspect. Download as PDF, TXT or read online. Dot Net 4.0 Programming Black Book. Dreamtech Press.NET 4.5 Programming (6 in 1) BB. Dreamtech Press.
- C# Programming Tutorial Pdf
- Programming C# 4.0 Pdf File
- Programming C# 4.0 Pdf
- Learning C# Programming Pdf
- Programming C# 4.0 Pdf Reader
The focus of this book is the C# language features that were introduced in versions 3.0, 4.0 and 5.0 For this book, you should know C# language programming. This book can be assumed as Part 2 of my previous book that covers C# language versions 1.0, 1.1 and some 2.0 features.
Fully updated for C# 4.0 and Visual Studio 2010, this eBook contains 28 chapters of detailed information designed to provide everything necessary to gain proficiency in the C# programming language and Visual Studio development environment.
The ebook begins with a detailed overview of the C# development and runtime environment including overviews of the Common Language Infrastructure (CLI), Common Intermediate Language (CIL) and Virtual Execution System (VES) followed by simple step by step guides to creating both Windows and console based C# applications.
A number of subsequent chapters are dedicated to the fundamentals of the C# programming language, covering variables, constants, operators, flow control, looping and object oriented programming (including areas such as inheritance and abstract classes). Later chapters focus on issues such as manipulating and formatting strings and working with arrays and collection classes.
Once the basics of the C# language have been covered the book then focuses on the design of GUI based applications using C# in conjunction with Visual Studio. Topics include the design of key GUI elements (such as forms, toolbars and menus) and a detailed overview of event handling in GUI based C# applications.
The two final chapters of the book are dedicated to the topic of drawing graphics in C#, including the use of bitmap images to create persistent graphics.
C# Programming Tutorial Pdf
On completing this C# eBook it is intended that the reader will have gained a firm knowledge foundation on which to begin developing complex C# based applications.
This purchase includes the eBook in both PDF and ePub formats.
Product Details
Page Count: 155Page Size: 8.50 x 11.00
Language: English
Format:
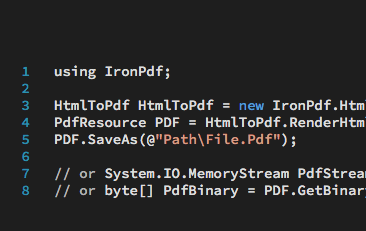
Price: $9.99
eBook Contents
- The C# Language and Environment
- A Simple C# Console Application
- Creating a Simple C# GUI Application with Visual Studio
- C# Variables and Constants
- C# Operators and Expressions
- C# Flow Control Using if and else
- The C# switch Statement
- C# Looping - The for Statement
- C# Looping with do and while Statements
- C# Object Oriented Programming
- C# Inheritance
- Understanding C# Abstract Classes
- Introducing C# Arrays
- C# List and ArrayList Collections
- Working with Strings in C#
- Formatting Strings in C#
- Working with Dates and Times in C#
- C# and Windows Forms
- Designing Forms in C# and Visual Studio
- Understanding C# GUI Events
- C# Events and Event Parameters
- Hiding and Showing Forms in C#
- Creating Top-Level Menus in C#
- Creating Context Menus in C#
- Building a Toolbar with C# and Visual Studio
- Drawing Graphics in C#
- Using Bitmaps for Persistent Graphics in C#
C# 4.0 is a version of the C# programming language that was released on April 11, 2010. Microsoft released the 4.0 runtime and development environment Visual Studio 2010.[1] The major focus of C# 4.0 is interoperability with partially or fully dynamically typed languages and frameworks, such as the Dynamic Language Runtime and COM.
Features[edit]
The following new features were added in C# 4.0.[2]
Dynamic member lookup[edit]
A new pseudo-type dynamic
is introduced into the C# type system. It is treated as System.Object
, but in addition, any member access (method call, field, property, or indexer access, or a delegate invocation) or application of an operator on a value of such type is permitted without any type checking, and its resolution is postponed until run-time. This is known as duck typing. For example:
Dynamic method calls are triggered by a value of type dynamic
as any implicit or explicit parameter (and not just a receiver). For example:
Dynamic lookup is performed using three distinct mechanisms: COM IDispatch for COM objects, IDynamicMetaObjectProvider
DLR interface for objects implementing that interface, and reflection for all other objects. Any C# class can therefore intercept dynamic calls on its instances by implementing IDynamicMetaObjectProvider
.
In case of dynamic method and indexer calls, overload resolution happens at run-time according to the actual types of the values passed as arguments, but otherwise according to the usual C# overloading resolution rules. Furthermore, in cases where the receiver in a dynamic call is not itself dynamic, run-time overload resolution will only consider the methods that are exposed on the declared compile-time type of the receiver. For example:
Any value returned from a dynamic member access is itself of type dynamic
. Values of type dynamic
are implicitly convertible both from and to any other type. In the code sample above this permits GetLength
function to treat the value returned by a call to Length
as an integer without any explicit cast. At run time the actual value will be converted to the requested type.
Covariant and contravariant generic type parameters[edit]
Generic interfaces and delegates can have their type parameters marked as covariant or contravariant using keywords out
and in
respectively. These declarations are then respected for type conversions, both implicit and explicit, and both compile time and run time. For example, the existing interface IEnumerable<T>
has been redefined as follows:
Therefore, any class that implements IEnumerable<Derived>
for some class Derived
is also considered to be compatible with IEnumerable<Base>
for all classes and interfaces Base
that Derived
extends, directly or indirectly. In practice, it makes it possible to write code such as:
Programming C# 4.0 Pdf File
For contravariance, the existing interface IComparer<T>
has been redefined as follows:
Therefore, any class that implements IComparer<Base>
for some class Base
is also considered to be compatible with IComparer<Derived>
for all classes and interfaces Derived
that are extended from Base
. It makes it possible to write code such as:
Optional ref keyword when using COM[edit]
The ref
keyword for callers of methods is now optional when calling into methods supplied by COM interfaces. Given a COM method with the signature
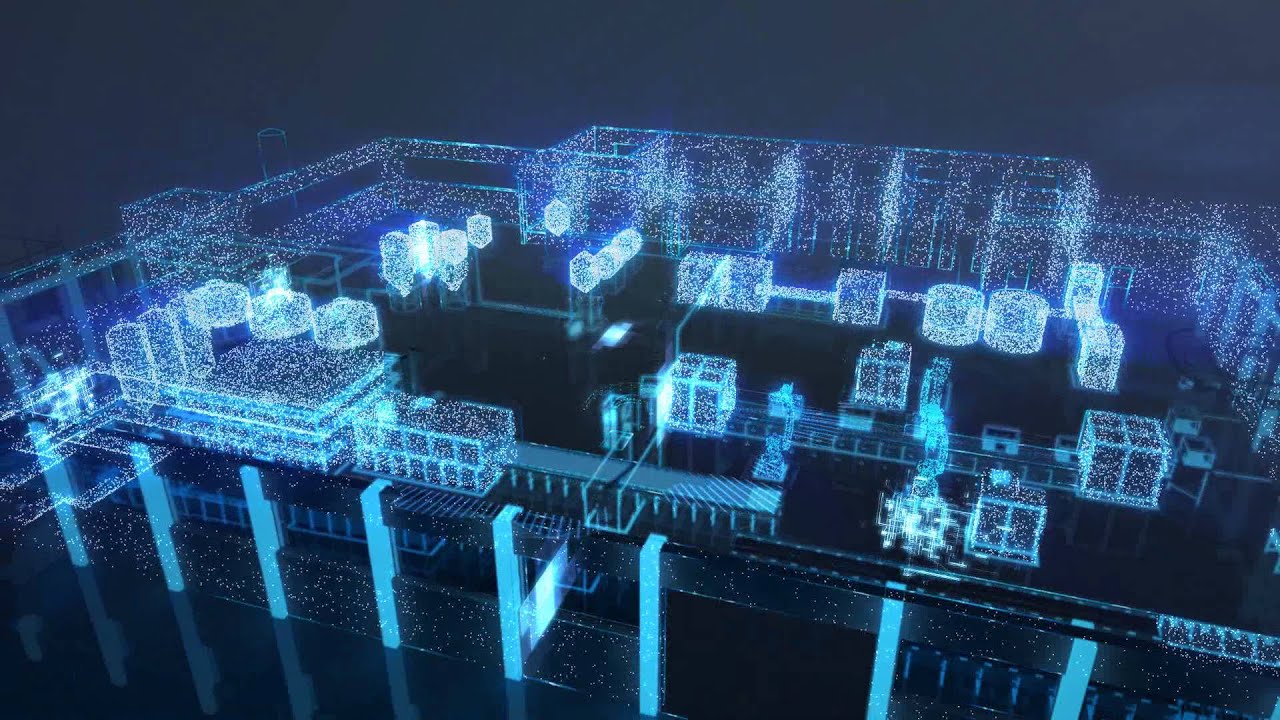
the invocation can now be written as either
or
Optional parameters and named arguments[edit]
C# 4.0 introduces optional parameters with default values as seen in Visual Basic and C++. For example:
In addition, to complement optional parameters, it is possible explicitly to specify parameter names in method calls, allowing the programmer selectively to pass any subset of optional parameters for a method. The only restriction is that named parameters must be placed after the unnamed parameters. Parameter names can be specified for both optional and required parameters, and can be used to improve readability or arbitrarily to reorder arguments in a call. For example:
Optional parameters make interoperating with COM easier. Previously, C# had to pass in every parameter in the method of the COM component, even those that are optional. For example:
With support for optional parameters, the code can be shortened as
Which, due to the now optional ref
keyword when using COM, can further be shortened as
Indexed properties[edit]
Programming C# 4.0 Pdf
Indexed properties (and default properties) of COM objects are now recognized, but C# objects still do not support them.
References[edit]
- ^'Microsoft Visual Studio 2010 First Look'.
- ^Torgersen, Mads (2008-10-27). 'New features in C# 4.0'. Microsoft. Retrieved 2008-10-28.
External links[edit]
Learning C# Programming Pdf
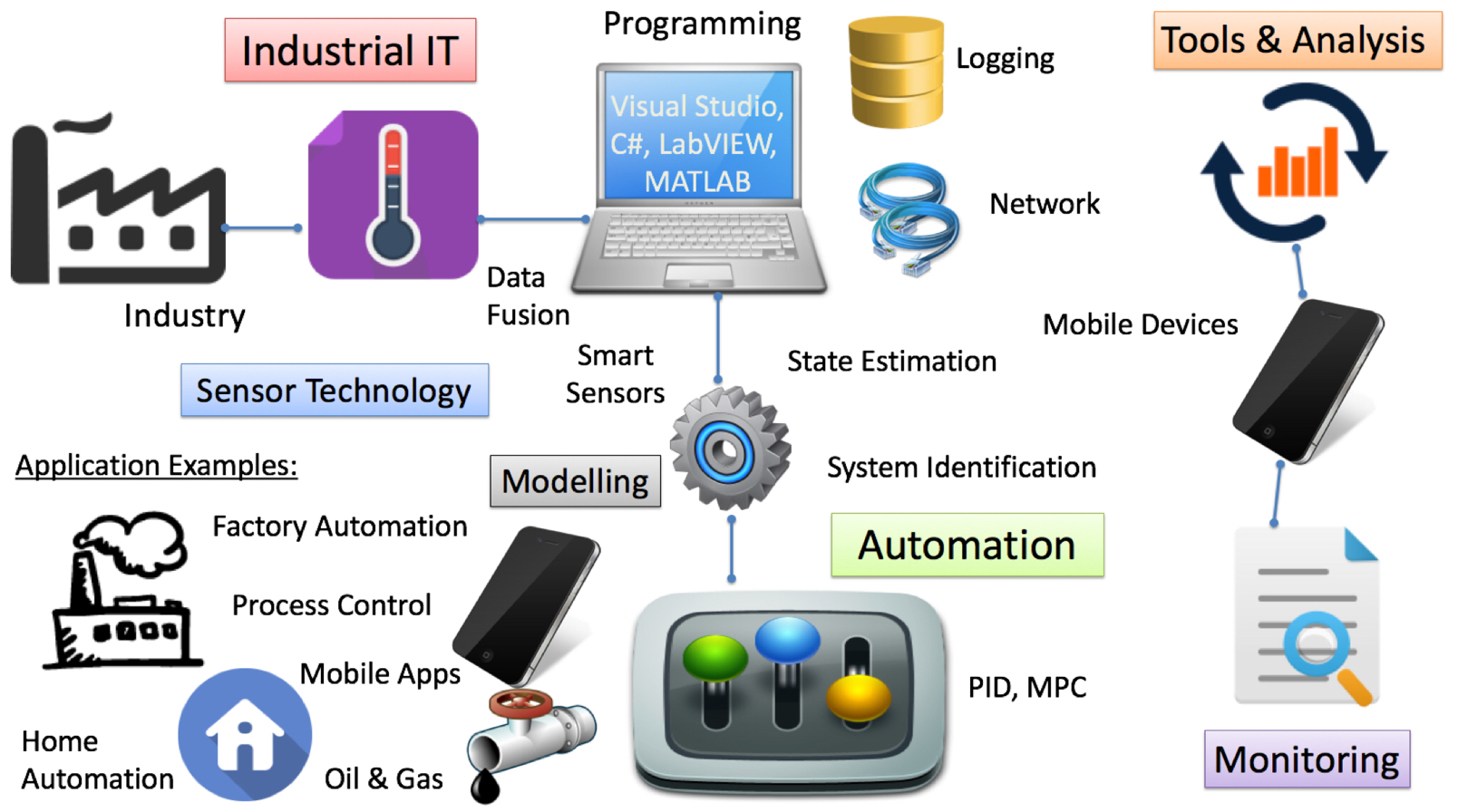